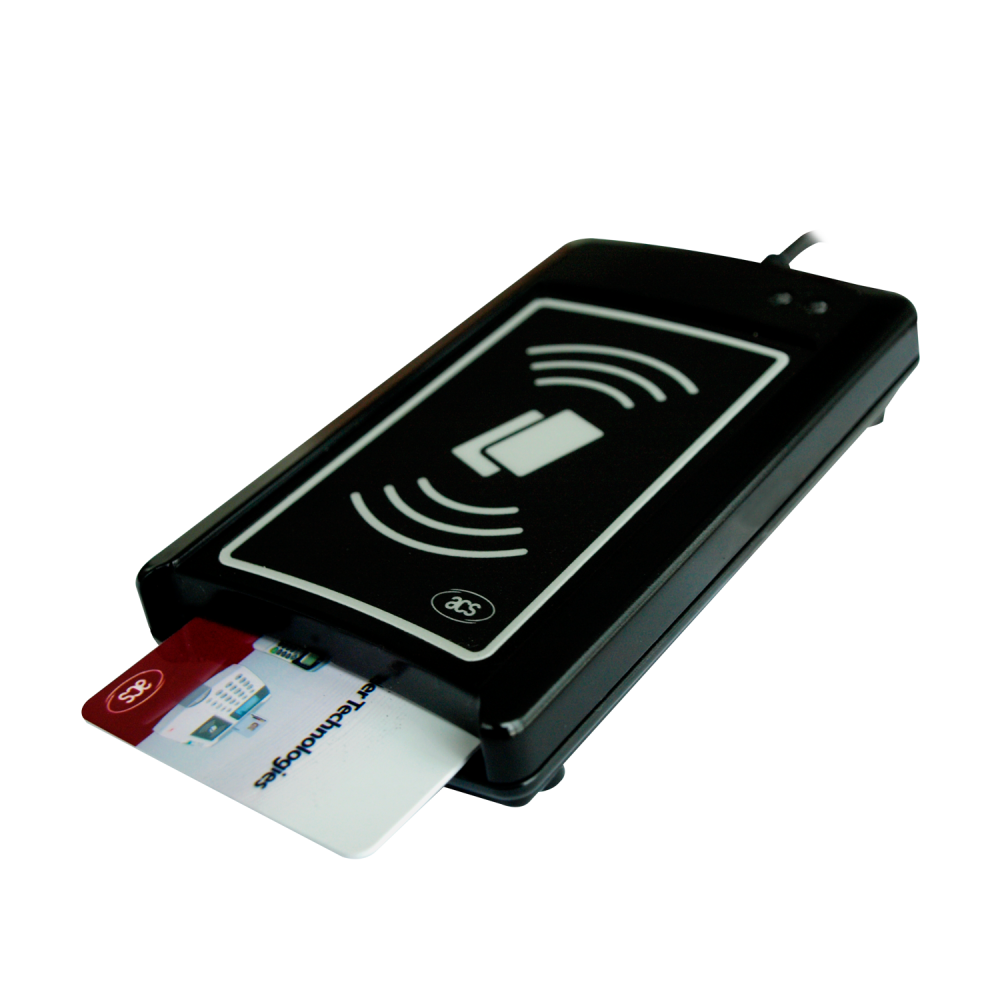
Android NFC Reader Mode: A Deep Dive
Explore Android NFC reader mode! Learn the communication protocol, code examples, and how to implement NFC tag reading in your Android applications.
Table of Contents
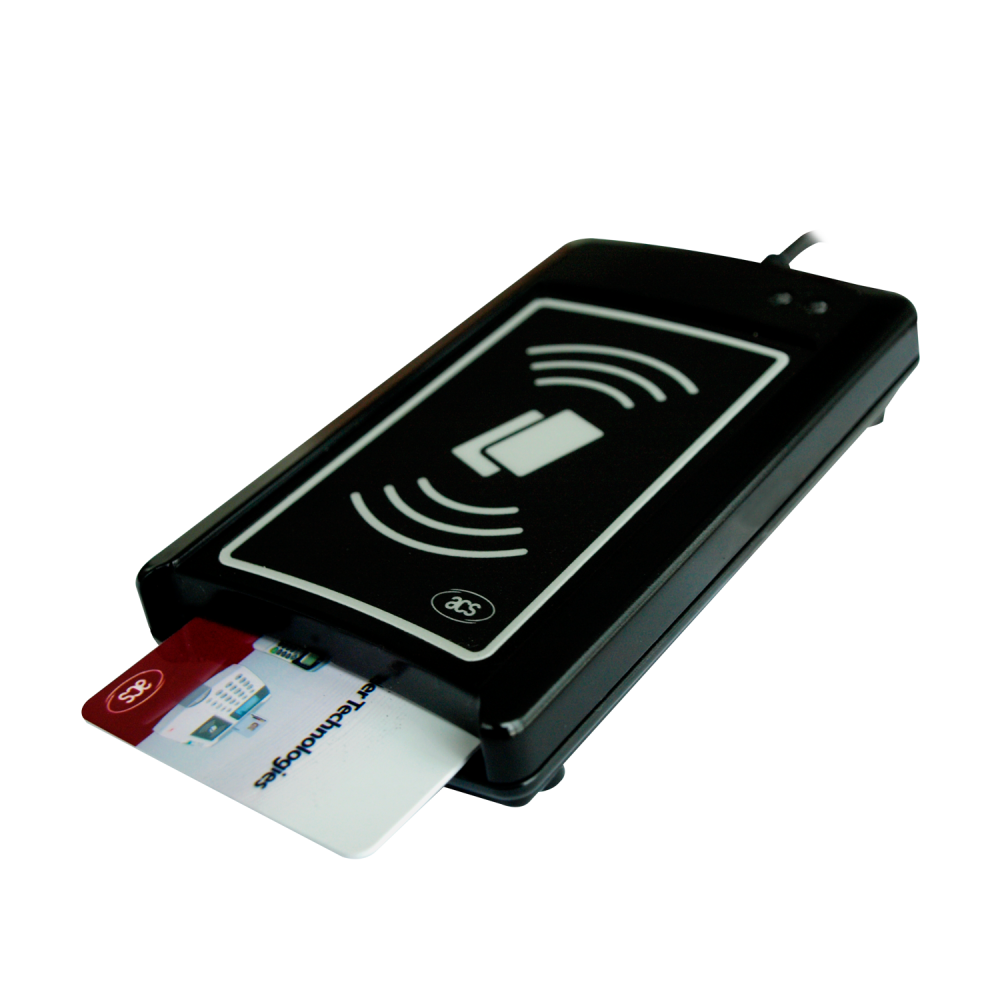
Android NFC Reader Mode: A Deep Dive
NFC (Near Field Communication) is a short-range wireless communication technology that enables data transfer between devices. On Android, NFC can be configured in various modes, and “Reader Mode” is a common use case. This post explores the Android NFC Reader Mode communication protocol, with code examples, to help developers understand its applications.
What is NFC Reader Mode?
In Reader Mode, an Android device can interact with other NFC-enabled devices like NFC tags and smart cards. By reading data, the Android device can retrieve information stored on the NFC tag, such as payment details, identification, or application data.
How NFC Reader Mode Works
NFC Reader Mode communication relies on a polling mechanism. The Android device actively searches for nearby NFC tags. When a tag is found, the device sends specific commands to read information. The tag then responds to the device’s request.
Communication Flow
This sequence diagram illustrates the Android NFC Reader Mode communication flow:
sequenceDiagram
participant NFC Tag
participant Android Device
Android Device->NFC Tag: Sends Polling Request
NFC Tag->Android Device: Returns Response Information
Android Device->NFC Tag: Sends Read Command
NFC Tag->Android Device: Returns Read Data
As shown, the Android device first sends a polling request, then sends a read command based on the returned information, and finally receives the data returned by the tag.
Android NFC Reader Mode Code Example
Implementing NFC Reader Mode in Android involves these steps:
1. Configure Permissions
Add the necessary permissions and feature declarations in AndroidManifest.xml:
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.nfcreader">
<uses-permission android:name="android.permission.NFC" />
<uses-feature android:name="android.hardware.nfc" android:required="true" />
<application
... >
<activity
android:name=".MainActivity"
... >
<intent-filter>
<action android:name="android.nfc.action.NDEF_DISCOVERED" />
<category android:name="android.intent.category.DEFAULT" />
<data android:mimeType="text/plain" />
</intent-filter>
</activity>
</application>
</manifest>
2. Enable NFC
Enable NFC in your Activity and handle NFC events:
import android.app.Activity;
import android.content.Intent;
import android.content.IntentFilter;
import android.nfc.NfcAdapter;
import android.nfc.Tag;
import android.nfc.tech.Ndef;
import android.os.Bundle;
import android.util.Log;
import android.app.PendingIntent;
public class MainActivity extends Activity {
private NfcAdapter nfcAdapter;
private PendingIntent pendingIntent;
private IntentFilter[] intentFilters;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
nfcAdapter = NfcAdapter.getDefaultAdapter(this);
if (nfcAdapter == null) {
Log.e("NFC", "This device doesn't support NFC");
finish();
return;
}
pendingIntent = PendingIntent.getActivity(this, 0, new Intent(this, getClass()).addFlags(Intent.FLAG_ACTIVITY_SINGLE_TOP), 0);
IntentFilter ndef = new IntentFilter(NfcAdapter.ACTION_NDEF_DISCOVERED);
try {
ndef.addDataType("text/plain"); // Handle MIME type
} catch (IntentFilter.MalformedMimeTypeException e) {
throw new RuntimeException("fail", e);
}
intentFilters = new IntentFilter[] {ndef};
}
@Override
protected void onResume() {
super.onResume();
if (nfcAdapter != null) {
nfcAdapter.enableForegroundDispatch(this, pendingIntent, intentFilters, null);
}
}
@Override
protected void onPause() {
super.onPause();
if (nfcAdapter != null) {
nfcAdapter.disableForegroundDispatch(this);
}
}
@Override
protected void onNewIntent(Intent intent) {
super.onNewIntent(intent);
if (NfcAdapter.ACTION_NDEF_DISCOVERED.equals(intent.getAction())) {
Tag tag = intent.getParcelableExtra(NfcAdapter.EXTRA_TAG);
readNfcTag(tag);
}
}
private void readNfcTag(Tag tag) {
// Read the tag data
// Read data based on tag type, e.g., Ndef
// ...
}
}
This code configures NFC permissions and demonstrates how to receive NFC tag events and handle read operations in an Activity.
3. Read NFC Tags
Reading NFC tags requires specific handling based on the tag type. Here’s a sample code:
private void readNfcTag(Tag tag) {
// Get Ndef technology
Ndef ndef = Ndef.get(tag);
if (ndef != null) {
try {
ndef.connect();
NdefMessage ndefMessage = ndef.getNdefMessage();
if (ndefMessage != null){
NdefRecord[] records = ndefMessage.getRecords();
for (NdefRecord record : records) {
String text = new String(new String(record.getPayload(), StandardCharsets.UTF_8)); // Corrected charset
Log.i("NFC Data", "Data read: " + text);
}
}
ndef.close();
} catch (Exception e) {
Log.e("NFC", "Error reading NFC tag", e);
}
}else {
Log.i("NFC", "NDEF not supported");
}
}
This example connects to the tag, reads records in its NDEF message, and extracts the stored information. Includes UTF-8 charset.
Common NFC Tag Types Distribution
pie
title NFC Tag Types Distribution
"NDEF Tags" : 40
"MIFARE Cards" : 30
"ISO 14443" : 20
"Other" : 10
This pie chart shows the common types of NFC tags and their distribution. Understanding different NFC tag types is crucial when implementing Reader Mode, as their reading/writing methods and data formats may differ.
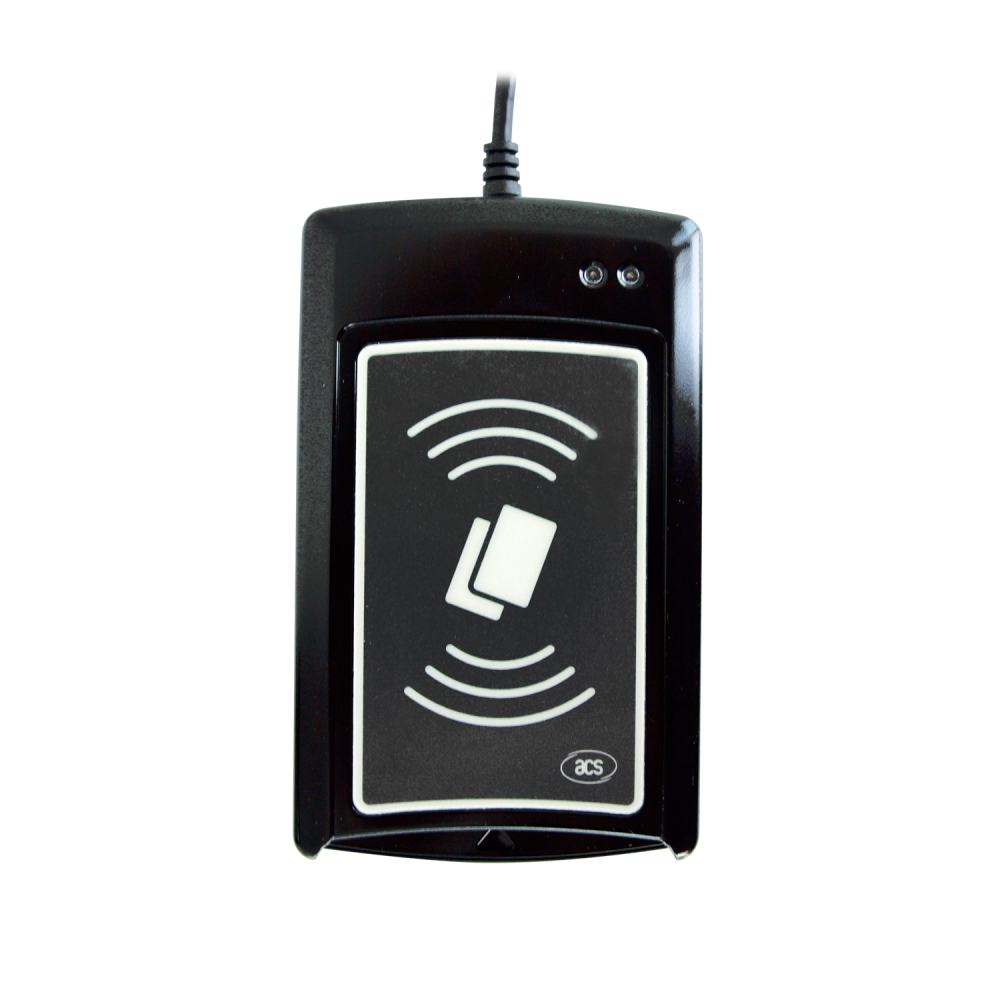
Conclusion
Android NFC Reader Mode is a powerful and flexible tool that provides developers with the ability to interact with various NFC devices. NFC technology has a wide range of applications, from payment systems to authentication and item tracking. With the code examples provided, you should be able to quickly get started and further explore the potential of NFC in mobile applications.