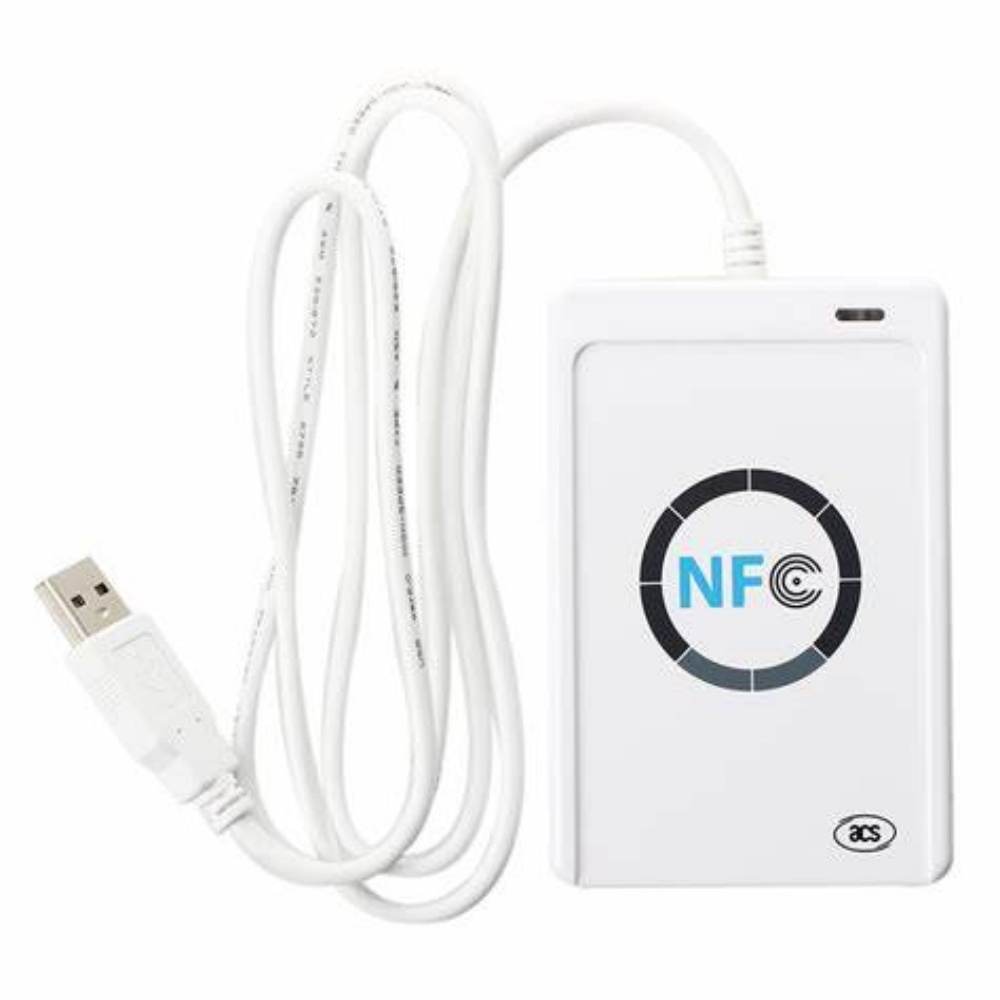
Web NFC Reader Integration
Integrating NFC Readers with web pages: Capturing card data, handling focus issues, and Javascript escaping. Ensure active browser & focused input for seamless data capture.
Table of Contents
Project Background
The goal is to read and process card information from a web page using a NFC reader connected to a computer.
Card Reader Data Output Process
- A card is placed near or on the card reader.
- The card reader parses the card information and formats it into a fixed-format string. This string includes data written to the card and original card data (ID, identifier, etc.). For example, an ID card might include name, ID number, card ID, and card identifier, with a generally standard data format.
- The card reader writes this string to the input field with focus on the current device (e.g., Word document, web page input tag).
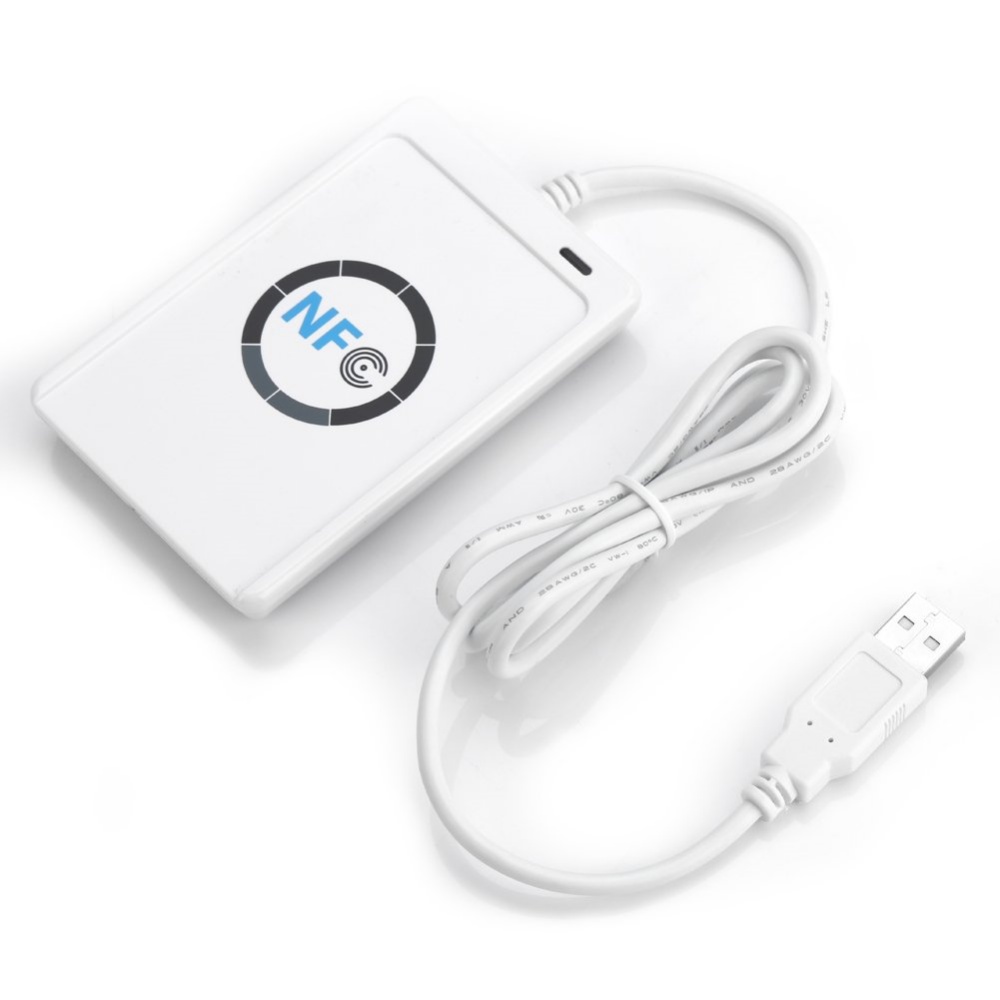
JavaScript String Escaping
The received string needs to be escaped for use in JavaScript.
function ascii2native( str ) {
return ( str + '' ).replace( /\\u[\da-z]{4}/gi , function( ascii ) {
return unescape( ascii.replace( /\\/, '%' ) ); // Name contains uppercase, convert to lowercase first
});
}
Note: Different card readers may vary. Some resources mention using decodeURIComponent (specifically for reading ID card names due to encoding differences). Choose the appropriate escaping method based on the device’s actual behavior.
Web Page Card Information Reception Process
- Add an input tag to the page to receive card information.
- 1.1 To hide the input tag, use positioning to move it off-screen or set the transparency to 0. Using the hidden attribute is reported to not work.
- Listen for the input event on the input tag and retrieve the value.
- Parse the card information and use it.
Important Considerations (Pitfalls)
I. Prerequisites for Receiving the String:
- The browser must be active (in the foreground). Card information is only sent to the foreground interface of the current device. The web page cannot guarantee it will always be active, so listen for window.blur and window.focus events to provide user prompts.
- The web page’s input tag must continuously have focus. Use a setInterval timer to ensure the input tag stays focused.
II. Scenarios Where Reception Fails Even When the Browser is Active:
- 3.1 The focus is in the address bar.
- 3.2 The focus is in the F12 developer tools or other plugin interfaces.
Key Point: Card information can only be successfully received when the input tag has focus and the interface focus is within the web page’s area.